Tag: wsei
C# – zajęcia 03-11-2016
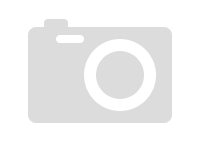
Materiał z dzisiejszych zajęć. Znajdziecie tutaj: QUIZ pętle foreach tablice (sortowanie, string jako tablica) losowanie liczb (liczb pseudolosowych, Sprawdzanie podzielności liczb) budowe listy(deklaracja i metoda Add())
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 |
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace zajecia031116 { class Program { static void Main(string[] args) { Random liczbaRandom = new Random(); //QUIZ int x = liczbaRandom.Next(1, 100); int y = 0; int krok = 1; Console.WriteLine("QUIZ - odgadnij liczbę"); Console.WriteLine(); Console.WriteLine(); do { if (krok == 11) { break; } Console.WriteLine("Podaj poszukiwaną liczbę. Krok: " + krok); y = Convert.ToInt16(Console.ReadLine()); if (y < x) { Console.WriteLine("Podana wartość jest za mała."); } else if (y > x) { Console.WriteLine("Podana wartość jest za duża."); } else if (y == x) { Console.WriteLine("Brawo!\nPrawidłowa wartość."); } krok++; } while (y != x); if (krok == 11) { Console.WriteLine("Przegrałeś! Wylosowana liczba to: " + x); } //LOSOWANIE Console.WriteLine("Losowanie 50 liczb pseudolosowych"); int v = 0; for (int i = 0; i < 50; i++) { x = liczbaRandom.Next(1, 1000); if (v % 2 == 0) { Console.WriteLine("Podzielna przez 2: {0}", v); } } Console.ReadLine(); //TABLICE int[] tablica = new int[3]; tablica[0] = liczbaRandom.Next(70, 200); tablica[1] = liczbaRandom.Next(2, 50); tablica[2] = liczbaRandom.Next(5, 100); int[] podzielne2 = new int[100]; int index2 = 0; int[] podzielne3 = new int[100]; int index3 = 0; int o = 0; for (int i = 0; i < 100; i++) { x = liczbaRandom.Next(1, 1000); if (o % 2 == 0) { podzielne2[index2] = o; index2++; } if (o % 3 == 0) { podzielne3[index3] = o; index3++; } } //Console.WriteLine("Podzielne przez 2, sposób pierwszy: "); //for (int i = 0; i < podzielne2.Length; i++) //{ // Console.WriteLine(podzielne2[i]); //} Console.WriteLine("Podzielne przez 2, sposób drugi: "); foreach (var liczba in podzielne2) { Console.WriteLine(liczba); } Console.WriteLine("Podzielne przez 3, foreach"); foreach (var liczba in podzielne3) { Console.WriteLine(liczba); } List<int> liczbyList = new List<int>(); liczbyList.Add(172); liczbyList.Add(21); liczbyList.Add(43); liczbyList.Add(24); if (liczbyList.Contains(23)) { Console.WriteLine("Zawiera 23."); } else { Console.WriteLine("Nie zawiera 23."); } Console.WriteLine("Elementów w liście: " + liczbyList.Count()); //string jako tablica char znak = 'a'; string zdanie = "Ala ma kota"; string zdanie2 = "kot ma Ale"; foreach (char c in zdanie) { Console.WriteLine(c); } //SORTOWANIE TABLICY Console.WriteLine("Przed"); foreach (var liczba in tablica) Console.WriteLine(liczba); Array.Sort(tablica); Console.WriteLine("Po"); foreach (var liczba in tablica) Console.WriteLine(liczba); Console.WriteLine(); Console.ReadLine(); } } } |
Jerzy KołakowskiPasjonat informatyki, bloger. Full-stack Developer Technologie: ASP.NET MVC ASP.NET…
Read More »C# – zajęcia 25-10-2016
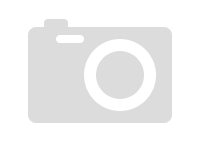
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
using System; using System.Collections.Generic; using System.Linq; using System.Runtime.InteropServices; using System.Text; using System.Threading.Tasks; namespace zajecia2 { class Program { static void Main(string[] args) { int k = 0; for (int i = 1; i <= 100; i++) { k = k + i; //to samo k+=i Console.WriteLine("Wartość k: " + k + "; Krok: " + i); } //do while int x = 11; do { Console.WriteLine(x); x++; } while (x <= 10); //while int i = 1; while (i <= 100) { Console.WriteLine(i); i++; } try { int a = 5; int b = 0; Console.WriteLine(a/b); } catch (DivideByZeroException exc) { Console.WriteLine(exc.Message); } catch (Exception ex) { Console.WriteLine(ex.Message); } int liczba1 = 0; int liczba2; bool isIntType; do { try { Console.WriteLine("Podaj 1 liczbę"); liczba1 = Convert.ToInt16(Console.ReadLine()); isIntType = false; } catch (Exception ex) { Console.WriteLine(ex.Message); isIntType = true; } } while (isIntType); Console.WriteLine("Podałeś liczbę: {0}", liczba1); } } } |
Jerzy KołakowskiPasjonat informatyki, bloger. Full-stack Developer Technologie: ASP.NET MVC ASP.NET CORE Angular 2+
Read More »